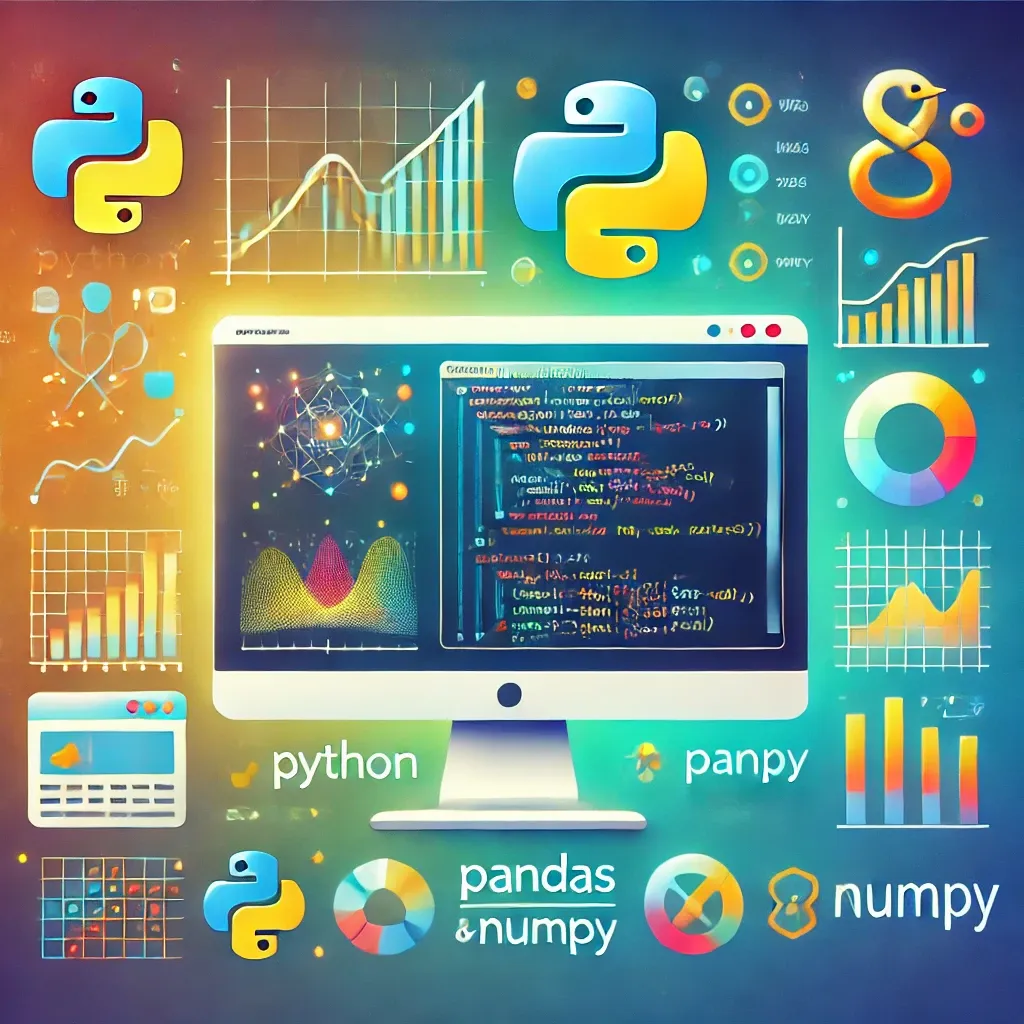
Introduction
In today’s data-driven world, knowing how to analyze and interpret data is crucial. Python, with its rich ecosystem of libraries, is widely used for data analysis, machine learning, and visualization. Among these libraries, Pandas and NumPy are indispensable tools for anyone dealing with datasets. This guide provides a beginner-friendly introduction to these libraries, covering their basic usage and showing how they make data manipulation easy.
What is Pandas?
Pandas is an open-source Python library designed for data manipulation and analysis. It provides powerful data structures such as DataFrames (two-dimensional data) and Series (one-dimensional data). These structures allow easy handling of tabular data and support a wide range of operations, including filtering, aggregation, and merging datasets.
What is NumPy?
NumPy (Numerical Python) is a foundational library used for working with numerical data. It introduces a new data type called arrays, which are faster and more efficient than Python lists. NumPy supports complex mathematical operations and is often used in scientific computing.
Setting Up the Environment
To get started, install Pandas and NumPy using the following commands:
pip install pandas numpy
You can verify the installation by importing the libraries in Python:
import pandas as pd import numpy as np
Working with NumPy Arrays
1. Creating NumPy Arrays
You can create arrays from lists or use built-in functions such as arange()
and ones()
:
import numpy as np # Array from a list array = np.array([1, 2, 3, 4]) # Array with a range of numbers range_array = np.arange(1, 10, 2) # Array filled with ones ones_array = np.ones((3, 3))
2. Basic Operations on Arrays
NumPy arrays support element-wise operations:
array = np.array([10, 20, 30, 40]) # Element-wise addition array += 5 # Element-wise multiplication result = array * 2
3. Array Statistics
You can calculate basic statistics using NumPy:
data = np.array([1, 2, 3, 4, 5]) print("Mean:", np.mean(data)) print("Standard Deviation:", np.std(data)) print("Sum:", np.sum(data))
Working with Pandas DataFrames
1. Creating a DataFrame
DataFrames in Pandas are similar to tables in databases or spreadsheets. You can create them from dictionaries or CSV files:
import pandas as pd # DataFrame from a dictionary data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 30, 35], 'Salary': [50000, 60000, 70000] } df = pd.DataFrame(data) print(df)
2. Reading Data from a CSV File
You can easily load external datasets using read_csv()
:
df = pd.read_csv('data.csv') print(df.head())
3. Filtering Data
Filtering rows based on conditions is simple with Pandas:
# Filter employees with Salary greater than 55000 filtered_df = df[df['Salary'] > 55000] print(filtered_df)
4. Adding and Removing Columns
You can add new columns or drop existing ones:
# Add a new column df['Bonus'] = df['Salary'] * 0.10 # Remove a column df.drop('Age', axis=1, inplace=True)
Data Aggregation and Grouping in Pandas
Pandas allows you to group data and perform aggregations:
# Group by 'Name' and calculate the average Salary grouped = df.groupby('Name')['Salary'].mean() print(grouped)
Handling Missing Data
Missing data is common in real-world datasets. Pandas provides several ways to handle it:
# Replace missing values with a default value df.fillna(0, inplace=True) # Drop rows with missing values df.dropna(inplace=True)
Merging and Joining DataFrames
You can merge multiple DataFrames using merge()
or concat()
:
df1 = pd.DataFrame({'ID': [1, 2], 'Name': ['Alice', 'Bob']}) df2 = pd.DataFrame({'ID': [1, 2], 'Salary': [50000, 60000]}) # Merge on 'ID' merged_df = pd.merge(df1, df2, on='ID') print(merged_df)
Visualizing Data with Pandas and Matplotlib
Pandas integrates with Matplotlib to create visualizations:
import matplotlib.pyplot as plt # Plot a histogram of salaries df['Salary'].plot(kind='hist', title='Salary Distribution') plt.show()
Conclusion
Pandas and NumPy are essential tools for anyone working with data in Python. They simplify the process of data manipulation, making it easy to clean, analyze, and visualize data. Whether you're a beginner or an experienced data scientist, mastering these libraries will greatly enhance your data analysis capabilities.
0 Comments